includes vs indexOf – Which Should you Use to Find Values in JavaScript Arrays?
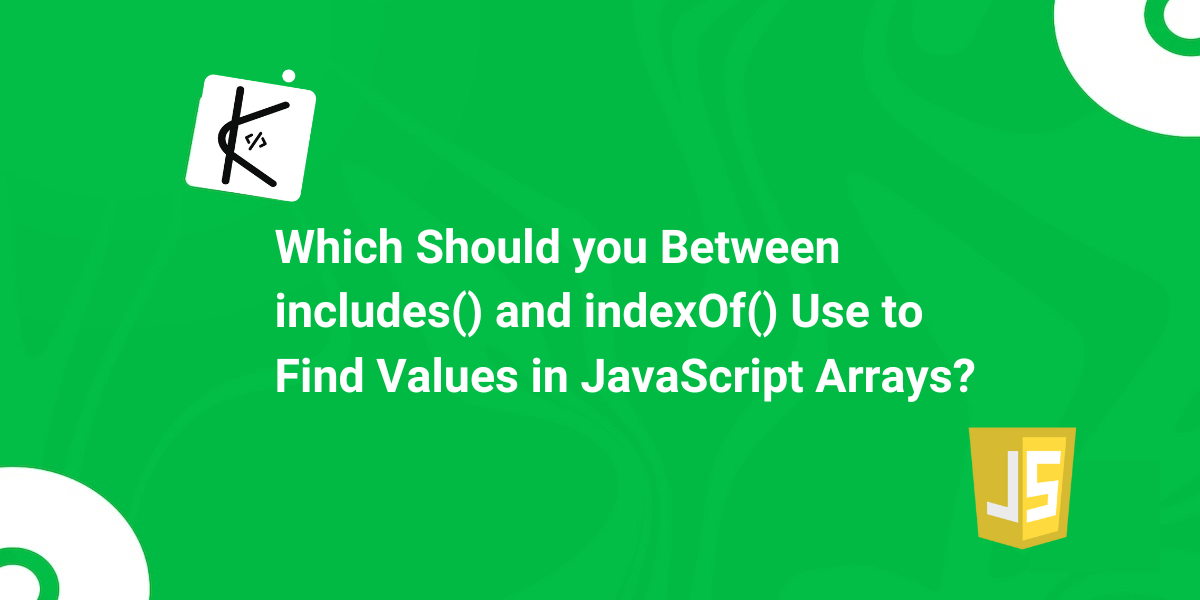
Written by Kolade Chris | May 18, 2025 | #JavaScript | 5 minutes Read
For a developer like you, finding values in JavaScript arrays is an everyday task. You might want to check if a user name exists in a list, or grab the position of a clicked item.
The two JavaScript methods that can help you do that are includes()
and indexOf()
. Choosing the right method between the two can go a long way in keeping your code clean and your intent clear.
In this article, you’ll learn how both includes()
and indexOf()
work, which of them to choose and when, and how that choice benefits you.
What is the includes()
Method and How Does it Work?
includes()
scans an array for a value and stops as soon as it finds a match. It uses strict equality (===
) to compare values and then returns a simple true
or false
value.
So, think of this method as a way to ask your array a simple yes-or-no question, like “Hey, do you have this specific value anywhere inside you?”
Here’s the basic syntax of the includes()
method:
array.includes(valueToFind, fromIndex);
Here’s an example:
const defensiveMidfielders = ['Kante', 'Makalele', 'Caicedo', 'Rice'];
// Do we have Kante?console.log(deliciousFruits.includes('banana')); // true
// Do we have Rodri?console.log(defensiveMidfielders.includes('Rodri')); // false
You can see that with includes()
, you get a clear boolean answer, which is perfect for conditional checks.
You can also check if a certain item exists in an array with mixed data types:
const mixedArr = [5, 'hello', NaN, true];
// Does it include NaN? Yes!console.log(mixedArr.includes(NaN)); // true
Now, let’s look at the indexOf()
method.
What is the indexOf()
Method and How Does it Work?
The indexOf()
method goes through each element in an array until it finds the value you’re looking for, then returns its position. If it doesn’t find it, you get –1
.
indexOf()
is a bit more about location, like asking your array, “Okay, if you have this value, where’s the first place I can find it?”.
Here’s the basic syntax of the indexOf()
method:
array.indexOf(valueToFind, fromIndex);
Here’s an example:
const moreDefensiveMidfielders = [ 'Viera', 'Mikel', 'Olofinjana', 'Viera', 'Roy Keane',];
// Where is the first Viera?console.log(moreDefensiveMidfielders.indexOf('Viera'));// 0 (because it;s the first item in the array)
// Where is Roy Keane?console.log(moreDefensiveMidfielders.indexOf('Roy Keane')); // 4
// Where is the second Viera?console.log(moreDefensiveMidfielders.indexOf('Viera', 2));// 3 (because it's at index 3)
// Where is Jay Jay Okocha?console.log(moreDefensiveMidfielders.indexOf('Jay Jay Okocha'));// -1 (not found)
You can see that indexOf()
gives you a number 0 or greater based on the position of the item found, or -1
if the item cannot be found in the array.
The key difference between includes()
and indexOf()
is that indexOf()
does not find NaN
.
If you have NaN
in your array and use indexOf(NaN)
, it will return -1
:
const values = [NaN, 'Seed', 'Code'];
console.log(values.indexOf(NaN)); // -1console.log(values.includes(NaN)); // true
Which should you Use Between indexOf()
and includes()
and When?
Choosing which to use to find an element in an array between indexOf()
and includes()
depends on what you need to do, or know.
Use includes()
when:
- you only need to know if a value exists
- you want clearer code
- you might be dealing with
NaN
. IfNaN
could potentially be in your array and you need to correctly check for its presence,includes()
is the way to go
Use indexOf()
when:
- you need the position of the item
- you need to check for the first occurrence and potentially find subsequent occurrences of an item
- you specifically need to support very, very old JavaScript environments that don’t have
includes()
Happy coding!